The read_excel
function from the Pandas library is a powerful tool for data manipulation and analysis. It allows you to easily import data from Excel files into a Pandas DataFrame, providing a seamless way to work with structured data. This function is particularly useful when dealing with large datasets or when you need to perform complex data transformations. In this blog post, we will explore the ins and outs of read_excel
, covering everything from its basic usage to advanced features and troubleshooting common issues.
Getting Started with read_excel
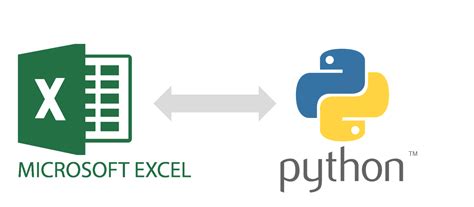
To begin, ensure you have the necessary packages installed. Pandas is a popular data analysis library in Python, and you can install it using the following command:
pip install pandas
Once Pandas is installed, you can import it into your Python script or notebook using the following line:
import pandas as pd
Now, let's dive into the basics of using read_excel
to load data from an Excel file into a Pandas DataFrame.
Basic Usage
The read_excel
function takes a few key arguments to specify the location of the Excel file and the desired sheet within it. Here's the basic syntax:
df = pd.read_excel(io, sheet_name=None, kwargs)
io
: This is the file path or URL of the Excel file you want to read. It can be a string or a path object.sheet_name
: Specifies the name of the sheet you want to read. If leftNone
, the first sheet will be selected.kwargs
: Additional keyword arguments that allow you to customize the reading process. We'll explore some of these later.
Let's look at a simple example where we read data from an Excel file named data.xlsx
located in the current working directory:
df = pd.read_excel('data.xlsx')
This will load the data from the first sheet of the Excel file into a Pandas DataFrame called df
. You can then explore and manipulate the data using various Pandas functions.
Specifying Sheet Names
If your Excel file contains multiple sheets, you can specify which sheet to read using the sheet_name
argument. Here's how you can do it:
df = pd.read_excel('data.xlsx', sheet_name='Sheet2')
In this example, 'Sheet2'
is the name of the sheet you want to read. You can also pass a list of sheet names to read multiple sheets at once.
Advanced Features and Customization
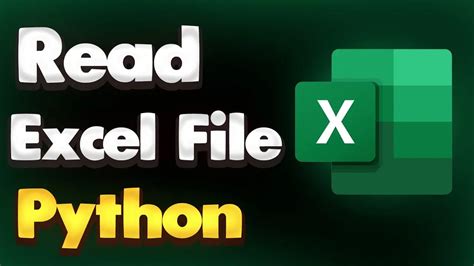
The read_excel
function offers a wide range of customization options to handle various data scenarios. Let's explore some of these advanced features.
Reading Specific Columns
If you only need specific columns from the Excel file, you can use the usecols
argument to specify them. This can significantly improve read performance, especially for large files.
df = pd.read_excel('data.xlsx', usecols=['Column1', 'Column2', 'Column3'])
In this example, only the specified columns will be read into the DataFrame.
Handling Data Types
By default, Pandas infers data types automatically. However, you can manually specify data types using the dtype
argument. This is particularly useful when dealing with non-standard data types or when you want to enforce specific data types.
df = pd.read_excel('data.xlsx', dtype={'Column1': 'category', 'Column2': 'float64'})
Here, we've specified that Column1
should be treated as a categorical variable and Column2
as a float.
Handling Missing Data
Pandas offers various options to handle missing data during the reading process. You can specify how to handle missing values using the na_values
argument. For example, to treat empty cells as missing values:
df = pd.read_excel('data.xlsx', na_values=['', '#N/A'])
Additionally, you can fill missing values with a specific value using the na_filter
argument:
df = pd.read_excel('data.xlsx', na_filter=False)
This will treat all cells with missing values as regular data, rather than filtering them out.
Skipping Rows and Columns
If your Excel file contains header rows or unnecessary columns, you can skip them using the skiprows
and skipfooter
arguments. For example, to skip the first 2 rows and the last 3 rows:
df = pd.read_excel('data.xlsx', skiprows=2, skipfooter=3)
You can also skip specific rows or columns by providing a list of row or column indices.
Handling Excel File Formats
Pandas supports reading various Excel file formats, including .xls
, .xlsx
, .xlsm
, and .xlsb
. The file format is automatically detected, but you can also specify it manually using the engine
argument. For example, to read an .xls
file:
df = pd.read_excel('data.xls', engine='xlrd')
Here, we've explicitly specified the xlrd
engine to read the .xls
file format.
Troubleshooting Common Issues
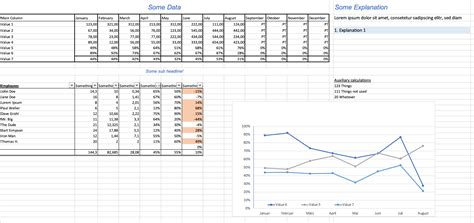
While read_excel
is a powerful tool, you might encounter some common issues when working with Excel files. Here are a few troubleshooting tips:
Error: No such file or directory
If you receive an error indicating that the file doesn't exist, ensure that the file path is correct and that the file is accessible. Double-check the file name and extension, as case sensitivity might be an issue.
Error: Invalid file format
If Pandas cannot detect the file format, ensure that you're using the correct engine for the file type. For example, xlrd
for .xls
files and openpyxl
for .xlsx
files.
Performance Issues
Reading large Excel files can be time-consuming. To improve performance, consider using the usecols
argument to read only the necessary columns and the nrows
argument to limit the number of rows read.
Handling Password-Protected Files
If your Excel file is password-protected, you'll need to provide the password when reading the file. You can do this using the password
argument:
df = pd.read_excel('data.xlsx', password='your_password')
Tips for Efficient Data Handling

To ensure smooth and efficient data handling, here are a few additional tips:
- Use the
index_col
argument to set a column as the index of the DataFrame. - If your Excel file has a header row, set
header=0
to use the first row as the column names. - For complex data transformations, consider using Pandas'
read_csv
function in conjunction withxlrd
to read Excel files as CSV.
Conclusion
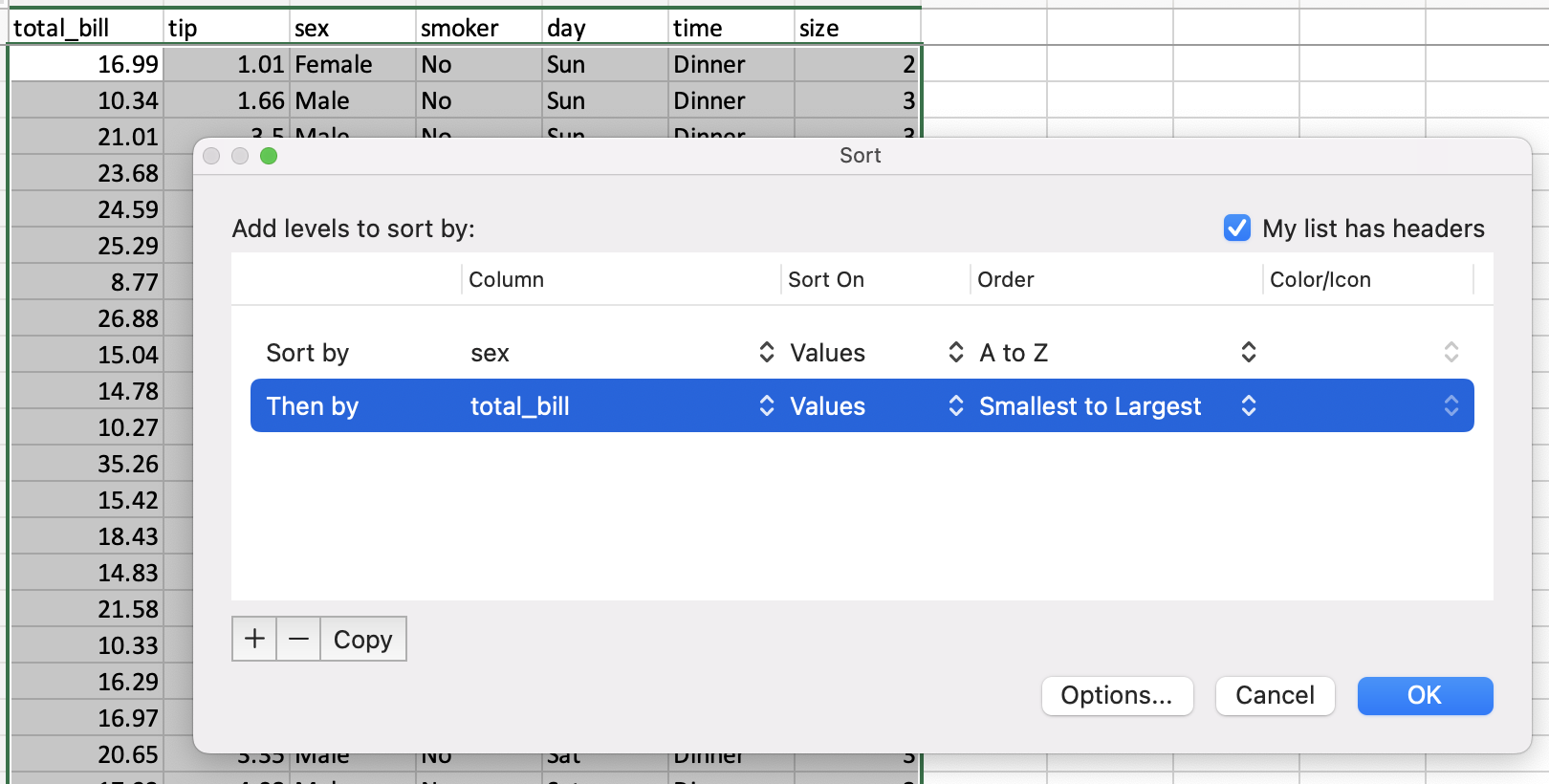
The read_excel
function from Pandas is a versatile tool for importing data from Excel files into Pandas DataFrames. With its wide range of customization options, you can efficiently handle various data scenarios and perform complex data transformations. Whether you're working with simple or complex Excel files, read_excel
provides the flexibility and power you need for your data analysis tasks.
FAQ

How can I read multiple sheets from an Excel file at once?
+You can read multiple sheets from an Excel file by passing a list of sheet names to the sheet_name
argument. For example, sheet_name=[‘Sheet1’, ‘Sheet2’]
will read data from both sheets.
Can I read Excel files directly from a URL?
+Yes, you can read Excel files from a URL by providing the URL as the io
argument. For example, io=’https://example.com/data.xlsx’
will read the Excel file from the specified URL.
How do I handle Excel files with merged cells?
+Excel files with merged cells can cause issues when reading data. To handle this, you can use the merge_cells=True
argument to preserve the merged cells during the reading process.
Is it possible to read Excel files with multiple worksheets?
+Yes, Pandas supports reading Excel files with multiple worksheets. You can specify the desired worksheet using the sheet_name
argument. If you want to read data from all worksheets, you can use the sheet_name=None
argument.
Can I read Excel files with special characters in their names?
+Yes, Pandas can handle Excel files with special characters in their names. However, ensure that the file path is correctly escaped to avoid any issues. For example, io=r’C:\Data\data.xlsx’
for a file named data.xlsx
with a space in the path.