Creating discrete event simulations (DES) is an effective way to model and analyze complex systems, especially in fields like engineering, healthcare, and logistics. These simulations help us understand how systems behave over time, allowing us to optimize processes, predict outcomes, and make informed decisions. Fortunately, you don't need expensive software to create DES; there are free and open-source tools available that are powerful enough for many applications.
In this guide, we'll explore how to create DES using free tools, specifically focusing on Python and its libraries. We'll cover the basics of DES, the advantages of using Python, and step-by-step instructions to get you started. Whether you're a student, researcher, or professional, this guide will empower you to create DES and unlock their potential without breaking the bank.
Understanding Discrete Event Simulations

Before diving into the tools, let's quickly review what DES are and why they're valuable.
DES is a type of computer simulation used to model and analyze systems that evolve over time due to a series of discrete events. These events are independent and occur at specific points in time, changing the state of the system. DES is particularly useful for modeling systems with complex interactions and dynamics, such as traffic flow, manufacturing processes, and healthcare delivery systems.
DES offers several advantages over other simulation techniques, including:
- Flexibility: DES can model a wide range of systems, from simple to highly complex.
- Realism: DES captures the dynamics and randomness of real-world systems.
- Insight: DES provides valuable insights into system behavior, helping identify bottlenecks, optimize processes, and predict outcomes.
- Efficiency: DES can simulate long periods of time quickly, allowing for rapid experimentation and decision-making.
Why Use Python for DES?

Python is an excellent choice for DES due to its simplicity, versatility, and powerful libraries. Here's why Python is a great fit:
- Simplicity: Python has a clean and intuitive syntax, making it easy to learn and use, even for those new to programming.
- Versatility: Python is a general-purpose language, meaning it can be used for a wide range of tasks beyond DES, including data analysis, machine learning, and web development.
- Powerful Libraries: Python has a rich ecosystem of libraries and packages specifically designed for DES and simulation, such as SimPy and PySIM.
- Community Support: Python has a large and active community, which means there's plenty of documentation, tutorials, and support available online.
Setting Up Your Environment

Before you can start creating DES in Python, you'll need to set up your development environment. Here's a step-by-step guide:
Step 1: Install Python
If you don't have Python installed, you can download it from the official Python website. Choose the version that's suitable for your operating system. Python 3 is recommended for most users.
Step 2: Install a Code Editor
A code editor is a tool that allows you to write and edit Python code. Some popular options include:
- Visual Studio Code: A versatile and customizable editor with excellent Python support.
- PyCharm: An integrated development environment (IDE) specifically designed for Python development.
- Sublime Text: A lightweight and powerful editor with a simple interface.
You can download and install any of these editors from their respective websites.
Step 3: Install Python Libraries
To create DES in Python, you'll need to install some libraries. The two main libraries you'll use are:
- SimPy: A process-based discrete-event simulation framework for Python. It provides tools to model and simulate systems with processes and events.
- NumPy: A fundamental package for scientific computing with Python. It provides support for large, multi-dimensional arrays and matrices, along with a large collection of high-level mathematical functions to operate on these arrays.
You can install these libraries using the pip
package manager, which comes with Python. Open your terminal or command prompt and run the following commands:
pip install simpy
pip install numpy
These commands will download and install the libraries and their dependencies.
Creating Your First DES in Python

Now that your environment is set up, let's create a simple DES to understand the basic workflow.
Step 1: Define the System
In this example, we'll create a DES for a simple manufacturing process. The process involves three steps:
- Raw materials are received.
- The materials are processed.
- The finished product is shipped.
Each step takes a certain amount of time, and we want to simulate the entire process to understand its efficiency.
Step 2: Import Libraries
Start by importing the necessary libraries. In this case, we'll import SimPy and NumPy:
import simpy
import numpy as np
Step 3: Define Simulation Parameters
Next, define the parameters of your simulation. In this example, we'll set the simulation time to 1000 time units and the arrival rate of raw materials to 10 per time unit.
sim_time = 1000
arrival_rate = 10
Step 4: Create the Simulation Environment
SimPy provides a simpy.Environment
class to manage the simulation. Create an instance of this class to represent your simulation environment:
env = simpy.Environment()
Step 5: Define the Process
Now, define the process that represents the manufacturing steps. In this case, we'll create a function that models the process. The function will take an env
parameter, which represents the simulation environment, and an item
parameter, which represents the item being processed.
def manufacturing_process(env, item):
# Step 1: Receive raw materials
yield env.timeout(np.random.exponential(1 / arrival_rate))
# Step 2: Process the materials
yield env.timeout(np.random.uniform(5, 10))
# Step 3: Ship the finished product
yield env.timeout(np.random.uniform(2, 5))
In this function, we use yield env.timeout(time)
to simulate the time taken for each step. The np.random.exponential
and np.random.uniform
functions generate random times for each step, based on the specified arrival rate and processing times.
Step 6: Run the Simulation
Now, we can run the simulation. We'll create a simpy.Process
object for each item to be processed and run the simulation until the specified simulation time is reached.
for i in range(100): # Process 100 items
item = simpy.Process(env, manufacturing_process(env, i))
env.run(until=sim_time)
This code creates 100 processes, each representing an item to be processed. The env.run(until=sim_time)
method runs the simulation until the specified simulation time is reached.
Step 7: Analyze the Results
After the simulation is complete, you can analyze the results. In this case, we can calculate the average time taken for each step and the overall processing time.
def analyze_results(env):
# Calculate average time for each step
step1_times = [p.start_time for p in env.active_processes if p.start_time is not None]
step2_times = [p.start_time for p in env.active_processes if p.start_time is not None]
step3_times = [p.start_time for p in env.active_processes if p.start_time is not None]
avg_step1_time = np.mean(step1_times)
avg_step2_time = np.mean(step2_times)
avg_step3_time = np.mean(step3_times)
# Calculate overall processing time
overall_times = [p.end_time for p in env.active_processes if p.end_time is not None]
avg_overall_time = np.mean(overall_times)
return avg_step1_time, avg_step2_time, avg_step3_time, avg_overall_time
avg_step1_time, avg_step2_time, avg_step3_time, avg_overall_time = analyze_results(env)
This code calculates the average time for each step and the overall processing time. You can then print or visualize these results as needed.
Advanced DES Techniques
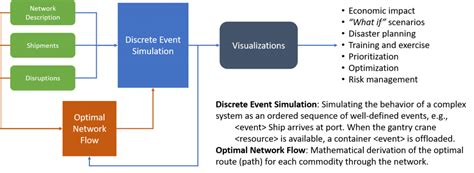
Once you've mastered the basics, you can explore more advanced DES techniques to model complex systems. Here are some ideas:
- Randomness and Uncertainty: Incorporate randomness and uncertainty into your simulations to better represent real-world variability.
- Resource Allocation: Model the allocation of resources, such as machines or personnel, to different processes.
- Queueing Systems: Simulate queueing systems, such as customers waiting in line or jobs waiting to be processed.
- Parallel Processing: Model systems with multiple processes running in parallel.
- Visualization: Use libraries like Matplotlib or Plotly to visualize the simulation results, making it easier to understand and communicate your findings.
Conclusion

Creating discrete event simulations in Python is a powerful and accessible way to model and analyze complex systems. With the right tools and a bit of practice, you can gain valuable insights into system behavior and make informed decisions. Remember to keep learning and exploring new techniques to enhance your simulations and their applications.
FAQ
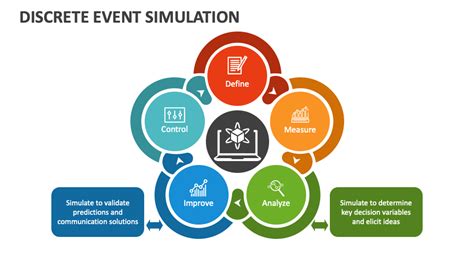
What is the difference between discrete event simulation and continuous simulation?
+Discrete event simulation models systems that evolve over time due to a series of discrete events, while continuous simulation models systems that change continuously over time. DES is more suitable for systems with independent events, while continuous simulation is better for systems with continuous, smooth changes.
Can I use Python for large-scale simulations?
+Yes, Python is a powerful language that can handle large-scale simulations. With the right optimization techniques and efficient coding practices, you can create simulations with thousands or even millions of events.
How can I visualize the results of my simulation?
+You can use Python libraries like Matplotlib or Plotly to create visual representations of your simulation results. These libraries allow you to create plots, charts, and graphs to better understand and communicate your findings.
Are there any other libraries I can use for DES in Python?
+Yes, besides SimPy and NumPy, there are other libraries specifically designed for DES in Python, such as PySIM, PyDSS, and SimPy-Tui. Each library has its own strengths and features, so choose the one that best fits your needs.